UCanCode
Software focuses on general application software development. We provide complete solution for developers. No matter you want to develop a simple database
workflow application, or an large flow/diagram based system, our product will provide a complete solution for you. Our product had been used by hundreds of top companies around the world!
"100% source code provided! Free you from not daring to use components because of unable to master the key technology of components!"
C# Article: Create, Open, Load, Save, Import,
Export, Edit, SVG File Document With C#, SVG .NET Class
Library
A simple C# application
and a C# class library
for editing SVG documents.
Introduction
This
article presents the
SVGPad application, a
simple C# tool designed
for editing SVG documents.
The SVGPad is a very simple editor
(it is not a WYSIWYG application) that can be useful for
learning SVG syntax and
for building simple SVG documents.
It provides a complete control over the
SVG document generated
(and this is not always true if you use a
graphic tool).
Background
The
article assumes that you
are familiar with basic concepts of
Scalable Vector Graphics (SVG),
an XML application that
provides a language to describe two-dimensional graphics.
SVG specification is published by W3C and can
be found here:
SVG graphics are based on three different
types of objects: vector graphics,
images, and text. Graphical objects
can be grouped, styled, transformed, and animated. Animation
is performed via scripting since a rich set of event
handlers (such as onmouseover, onclick, …) can be assigned
to any SVG object.
SVG has several benefits that make it the best
choice for web applications that need
2D graphic support. Because it is a
vector format the
graphic files are
smaller and they are resolution independent. In addition
since graphics are stored in a text file labels and
descriptions can be indexed by search engines.
SVGPad
How it works
The SVGPad UI is
divided in two main panes.
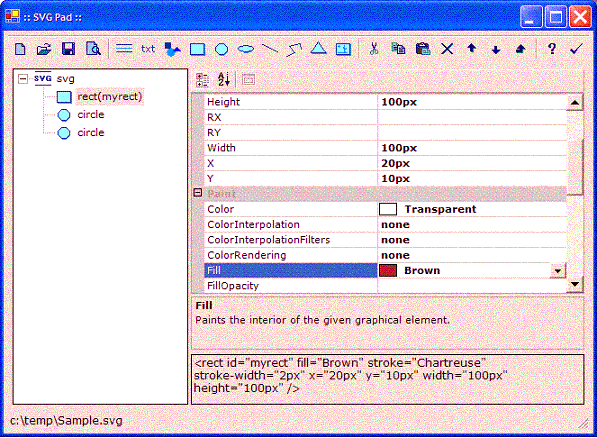
The left pane contains
a tree that represents the SVG
document. The right pane contains a PropertyGrid
displaying the property of the current selected object on
the tree and a text box that shows the
SVG representation of the selected object. The
PropertyGrid shows only the attributes that are suitable for
the element type selected.
The application
functionality can be accessed using the toolbar of via the
tree context menu.
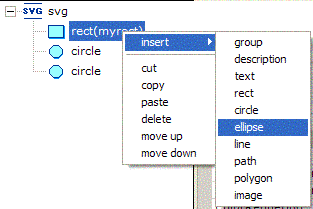
Supported
elements and attributes
The SVGPad does not
cover all the SVG
elements and attributes; in this first release it just
implements the most common elements:
-
text
-
desc
-
group
-
rect
-
circle
-
ellipse
-
line
-
path
-
polygon
-
image
For each element a
subset of SVG attributes is
supported:
-
Core attributes
-
Paint/Color
attributes
-
Style attributes
-
Opacity attributes
-
Graphic attributes
Other SVGPad
functions
-
New,
create a new empty
SVG document.
-
Open,
load an
SVG document from a
file.
-
Save,
save the current
document.
-
Preview, open the
SVG file in the
default web browser.
-
Copy, copy the
selected SVG element.
-
Cut, cut the
selected SVG
element.
-
Paste, paste the
element in the clipboard.
-
Delete, delete the
selected SVG
element.
-
Element up, move
the selected element up (before its previous element)
-
Element down, move
the selected element down (after its next element)
-
Level up, move the
selected element up one level in a hierarchy
SVGLib
The SVGPad application
is based on the SVGLib C# class
library. The SVGLib provides a set of classes
that represent the SVG document,
the SVG elements and
attributes. It can be used for creating and managing SVG
documents.
The SVGLib classes have
been documented using NDoc and the chm file is included in
the attached binary file zip.
Using the SVGLib is
very simple; first of all you need to create a
document:
SvgDoc doc = new SvgDoc();
SvgRoot root = doc.CreateNewDocument();
root.Width = "500px";
root.Height = "500px";
Then you can start
adding elements:
SvgRect rect = new SvgRect(doc,
"50px",
"25px",
"300px",
"200px",
"3px",
Color.LightPink,
Color.Black);
doc.AddElement(root, rect);
rect.RX = "2px";
rect.RY = "2px";
SvgCircle circle1 = new SvgCircle(doc,
"350px",
"200px",
"50px");
doc.AddElement(root, circle1);
circle1.Fill = Color.DarkBlue;
SvgCircle circle2 = new SvgCircle(doc,
"50px",
"200px",
"50px");
doc.AddElement(root, circle2);
circle2.Fill = Color.DarkBlue;
Finally you can
save the
document.
doc.SaveToFile("c:\\temp\\sampledoc.svg");
Here the created file
shown in a web browser.
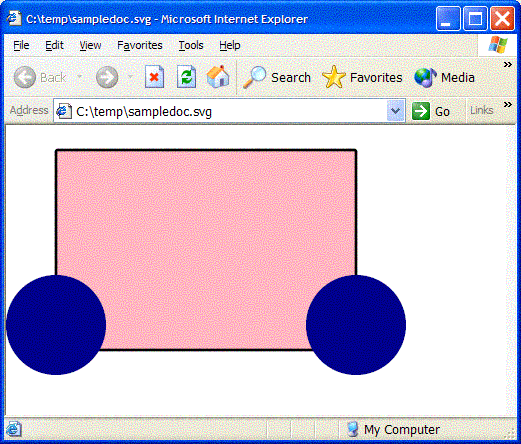
|