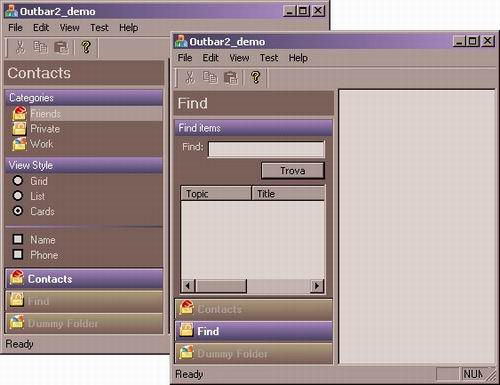
Introduction
This is a simple control
that, much like the outlook
side bar, docks on
the left side of your main window. Basically consists in
several folders, containing groups of items. Those items
can be either child windows or labels connected to the
standard menu command's ID.
Their appearance can be
customized so to act like rows in a list box, check
boxes, radio buttons, or simple links. All the actions
and the status of those items is controlled by the
standard MFC command
handler and command update handler.
Background
I have never seen the
latest outlook beside for one, small, screenshot; so I
don't really know if this control
resembles its properly or not.
I tried to include
support for all I saw in this screenshot, but I'm sure
much will still be different or wrong. It has been
written for use in one of our internal applications, but
.. since I found it cute, I thought maybe someone else
could've been interested into it. The control also works
well along with the BCGSoft
library, if you are using it, without needing
any change.
Using the code
The control
itself consist in 2 files, outlook2ctrl.h and .cpp.
To use it in your code, start by including those 2 files
in the project. Also, if you wish it to make use of the
gradient drawings on the buttons, change the WINVER
define in the project stdafx.h file from 0x400 to
0x500.
If WINVER
is below 0x500, the graphic won't look as good. (0x500
will mean compiled for Win 2K & XP).
Include in your main
frame header file the new control
in this way:
#include "Outlook2Ctrl.h"
class CMainFrame : public CFrameWnd
{
COutlook2Ctrl wndOutBar;
The outbar is derived
from the standard MGC CControlBar
(or from the CBCGToolBar
) and docks
normally on the left side of the main frame.
The CMainFrame::OnCreate
is a good place to create and initialize it:
Collapse
int CMainFrame::OnCreate(LPCREATESTRUCT lpCreateStruct)
{
...
EnableDocking(CBRS_ALIGN_TOP | CBRS_ALIGN_BOTTOM | CBRS_ALIGN_RIGHT);
wndOutBar.Create(this, ID_MYOUTBAR);
HICON hIco1 = (HICON) LoadImage(AfxGetInstanceHandle(),
MAKEINTRESOURCE(IDI_ICON1),IMAGE_ICON,16,16,0);
HICON hIco2 = (HICON) LoadImage(AfxGetInstanceHandle(),
MAKEINTRESOURCE(IDI_ICON2),IMAGE_ICON,16,16,0);
HICON hIco3 = (HICON) LoadImage(AfxGetInstanceHandle(),
MAKEINTRESOURCE(IDI_ICON3),IMAGE_ICON,16,16,0);
wndOutBar.AddFolderRes("Contacts", IDI_ICON1);
wndOutBar.AddFolderItem("Categories");
wndOutBar.AddSubItem("Friends", hIco1,
COutlook2Ctrl::OCL_SELECT, ID_CATEGORIES_FRIEND);
wndOutBar.AddSubItem("Private", hIco2,
COutlook2Ctrl::OCL_SELECT, ID_CATEGORIES_PRIVATE);
wndOutBar.AddSubItem("Work", hIco3,
COutlook2Ctrl::OCL_SELECT, ID_CATEGORIES_WORK);
wndOutBar.AddFolderItem("View Style");
wndOutBar.AddSubItem("Grid", NULL,
COutlook2Ctrl::OCL_RADIO, ID_VIEW_GRID);
wndOutBar.AddSubItem("List", NULL,
COutlook2Ctrl::OCL_RADIO, ID_VIEW_LIST);
wndOutBar.AddSubItem("Cards", NULL,
COutlook2Ctrl::OCL_RADIO, ID_VIEW_CARDS);
wndOutBar.AddFolderItem("");
wndOutBar.AddSubItem("Name",
NULL, COutlook2Ctrl::OCL_CHECK, ID_FIELDS_NAME);
wndOutBar.AddSubItem("Phone",
NULL, COutlook2Ctrl::OCL_CHECK, ID_FIELDS_PHONE);
wndOutBar.AddSubItem("Address",
NULL, COutlook2Ctrl::OCL_CHECK, ID_FIELDS_ADDRESS);
wndOutBar.AddFolderItem("");
wndOutBar.AddSubItem("Create new contact ..",
NULL, COutlook2Ctrl::OCL_COMMAND, ID_TEST_CREATENEWCONTACT);
wndOutBar.AddSubItem("Import contacts ..",
NULL, COutlook2Ctrl::OCL_COMMAND, ID_TEST_IMPORTCONTACT);
In this code,
we do a simple window creating and we then insert a few
items. The control
stores the data in a tree-like way. The base of the tree
is made by Folders
items (like the Contact
,
Find
, etc buttons you can see in the
image). The Folders
contain Items
- which are sort of groups; and they contain Subitem
s
that are what actually doing the job. So you insert a Folder
,
an Item
, and assign Subitem
s
to that.
The Subitem
s
are handled as if they were items of a menu. They are
checked/unchecked by using the standard CCmdUI
's
system. Selecting them will cause a menu command to be
generated, if appropriate. So it's basically a permanent
popup menu with a new graphical style, where Folders
are popups items, Subitems
are the menu
items, and Items
are description labels.
wndOutBar.AddFolderRes("Contacts", IDI_ICON1);
wndOutBar.AddFolderItem("Categories");
wndOutBar.AddSubItem("Friends",
hIco1, COutlook2Ctrl::OCL_SELECT, ID_CATEGORIES_FRIEND);
This part adds first a
folder, named Contact
, with icon IDI_ICON1
as graphic. It then adds a label named Categories
to the folder, and adds a menu command named Friends
that will trigger a menu command ID_CATEGORIES_FRIEND
when clicked. The subitem
will have a small
item on the side (with handle hIco1
) and
will be of type OCL_SELECT
.
The "types"
available are the following:
COutlook2Ctrl::OCL_SELECT
: the subitem
will appear as a selected
listbox item when the OnCmdUI
of the commandID
returns Checked
COutlook2Ctrl::OCL_RADIO
: the subitem
will appear as a radio
button; status will be determined by the
checked/unchecked of the associated commandID
;
no command will be sent when user clicks on an
already selected item
COutlook2Ctrl::OCL_COMMAND
: the subitem
will appear as a normal
hot-tracked link. The commandID
command
will be sent whenever user clicks on it, unless the OnCmdUI
returns not enabled
COutlook2Ctrl::OCL_CHECK
: the subitem
will appear as a check
box; status will be determined by the
checked/unchecked of the associated commandID
;
no command will be sent when user clicks on an
already selected item
COutlook2Ctrl::OCL_HWND
: this value is settled automatically when using the
following function:
int AddSubItem(HWND hChildWindow,
bool bStretchTheChild, int FolderId, int ItemId)
Takes in the child
window to insert, a stretch option value and the
folder and item ID. The child window has to be
already created when inserted. It has to have as
parent, the outlook
bar control, and
the WS_CHILD
flag. If the stretch
variable isn't settled, the child will retain the
value it had at creation; otherwise, the height of
the child window will be enlarged to fit all the
area from insertion to the bottom of control.
The child window will anyway always be stretched to
cover all the width of the outlook
bar control. The FolderID
and ItemID
variables in all the
insertion functions by default will be settled to
-1, and thus referring to the "last"
parent inserted.
The other functions for
inserting items are the following:
int AddFolder(const char * FolderName,
HICON ImageofFolder or int ResourceIdofFolderIcon);
int AddFolderItem(const char * ItemName, int FolderID = -1)
int AddSubItem(const char * SubItemLabel,
HICON ImageofFolder or int ResourceIdofFolderIcon,
DWORD style, DWORD commandID, int FolderId, int ItemId)
The const char
*
parameters are the labels of the item
s/folder
s/subitem
.
The HICON handles
or IDs are used for the
images linked to the folder
s/ subitem
s.
Note that some kind of subitem
s can't have
an icon linked to them (checkbox and radio box style).
Those functions all return the index of the inserted
element. You can use it for inserting other elements
into the same folder, or just leave them to the default
-1 to add them to the last one.
When a subitem
will be selected, you will receive a normal menu
command.
Points of interest
The control
has been tested just under Windows 2000 and XP. It
should work even under earlier versions of Windows, but
some resource memory leak may be possible. It has
nothing really amazing in it, but is simple to use and
cute to watch (I hope!). Enjoy it then!