Lines,
CDC, MoveTo, LineTo
|
|
A
line is a junction of two points. This means that
a line has a beginning and an end:
The
beginning and the end are two distinct points that
can be either POINT, CPoint,
or a mix of a POINT and
a CPoint values. In
real life, before drawing, you should define where
you would start. To help with this, the CDC
class provides the MoveTo()
method. It comes in two versions that are:
CPoint MoveTo(int x, int y);
CPoint MoveTo(POINT point);
|
The
origin of a drawing is specified as a CPoint
value. You can provide its horizontal and vertical
measures as x and y or you can pass it as a POINT
or a CPoint value.
To
end the line, you use the CDC::LineTo()
method. It comes it two versions declared as
follows:
BOOL LineTo(int x, int y);
BOOL LineTo(POINT point);
The
end of a line can be defined by its horizontal (x)
and its vertical measures (y). It can also be
provided as a POINT or
a CPoint value. The
last point of a line is not part of the line. The
line ends just before reaching that point.
Here
is an example that draws a line starting at a
point defined as (10, 22) coordinates and ending
at (155, 64):
void CExoView::OnDraw(CDC* pDC)
{
pDC->MoveTo(10, 22);
pDC->LineTo(155, 64);
}
|
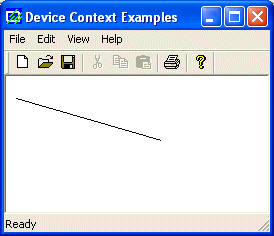 |
We
have mentioned that the CDC::MoveTo()
method is used to set the starting position of a
line. When using LineTo(),
the line would start from the MoveTo()
point to the LineTo()
end. As long as you do not call MoveTo(),
any subsequent call to LineTo()
would draw a line from the previous LineTo()
to the new LineTo()
point. You can use this property of the LineTo()
method to draw various lines. Here is an example:
void CExoView::OnDraw(CDC* pDC)
{
pDC->MoveTo(60, 20);
pDC->LineTo(60, 122);
pDC->LineTo(264, 122);
pDC->LineTo(60, 20);
}
|
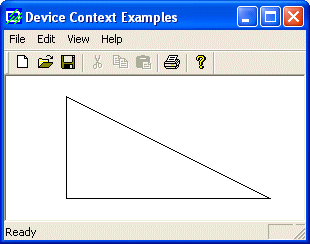 |
Practical Learning: Drawing Lines
|
|
- Start
Microsoft Visual C++ and create a Single
Document application called GDI1 and based on
the CView class
- Access
the OnPaint event of your view class and
implement it as follows:
void CView1View::OnPaint()
{
CPaintDC dc(this); // device context for painting
// TODO: Add your message handler code here
CPoint PtLine[] = { CPoint( 50, 50), CPoint(670, 50),
CPoint(670, 310), CPoint(490, 310),
CPoint(490, 390), CPoint(220, 390),
CPoint(220, 310), CPoint( 50, 310),
CPoint( 50, 50) };
dc.MoveTo(PtLine[0]);
dc.LineTo(PtLine[1]);
dc.LineTo(PtLine[2]);
dc.LineTo(PtLine[3]);
dc.LineTo(PtLine[4]);
dc.LineTo(PtLine[5]);
dc.LineTo(PtLine[6]);
dc.LineTo(PtLine[7]);
dc.LineTo(PtLine[8]);
// Do not call CView::OnPaint() for painting messages
}
|
- Test
the application
|