GDI
Topics: PolyPolygon, Polyline, Polygon
|
|
The
polylines we have used so far were drawn by
defining the starting point of the first line and
the end point of the last line and there was no
relationship or connection between these two
extreme points. A polygon is a closed polyline. In
other words, it is a polyline defined so that the
end point of the last line is connected to the
start point of the first line.
To
draw a polygon, you can use the CDC::Polygon()
method. Its syntax is:
BOOL Polygon(LPPOINT lpPoints, int nCount);
|
This
member function uses the same types of arguments
as the Polyline() method. The only difference is
on the drawing of the line combination. Here is an
example:
void CExoView::OnDraw(CDC* pDC)
{
CPoint Pt[7];
Pt[0] = CPoint(20, 50);
Pt[1] = CPoint(180, 50);
Pt[2] = CPoint(180, 20);
Pt[3] = CPoint(230, 70);
Pt[4] = CPoint(180, 120);
Pt[5] = CPoint(180, 90);
Pt[6] = CPoint(20, 90);
pDC->Polygon(Pt, 7);
}
|
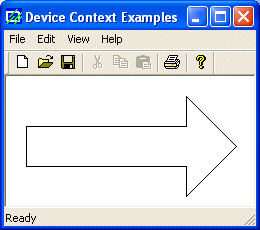 |
Practical Learning: Drawing Polygons
|
|
- To draw some polygons, change the program as follows:
void CView1View::OnPaint()
{
CPaintDC dc(this); // device context for painting
// TODO: Add your message handler code here
CPoint PtLine[] = { CPoint( 50, 50), CPoint(670, 50),
CPoint(670, 310), CPoint(490, 310),
CPoint(490, 390), CPoint(220, 390),
CPoint(220, 310), CPoint( 50, 310),
CPoint( 50, 50) };
CPoint Bedroom1[] = { CPoint( 55, 55), CPoint(175, 55),
CPoint(175, 145), CPoint( 55, 145)};
CPoint Closets[] = { CPoint( 55, 150), CPoint(145, 150),
CPoint(145, 205), CPoint( 55, 205) };
CPoint Bedroom2[] = { CPoint(55, 210), CPoint(160, 210),
CPoint(160, 305), CPoint(55, 305) };
dc.MoveTo(PtLine[0]);
dc.LineTo(PtLine[1]);
dc.LineTo(PtLine[2]);
dc.LineTo(PtLine[3]);
dc.LineTo(PtLine[4]);
dc.LineTo(PtLine[5]);
dc.LineTo(PtLine[6]);
dc.LineTo(PtLine[7]);
dc.LineTo(PtLine[8]);
dc.Polygon(Bedroom1, 4);
dc.Polygon(Closets, 4);
dc.Polygon(Bedroom2, 4);
// Do not call CView::OnPaint() for painting messages
}
|
- Test the
application and return to MSVC
If
you want to draw multiple polygons, you can use
the CDC::PolyPolygon() method whose syntax is:
BOOL PolyPolygon(LPPOINT lpPoints, LPINT lpPolyCounts, int nCount);
Like
the Polygon() method, the lpPoints
argument is an array of POINT or CPoint
values. The PolyPolygon() method needs to
know the number of polygons you would be drawing.
Each polygon uses the points of the lpPoints
values but when creating the array of points, the
values must be incremental. This means that PolyPolygon()
will not randomly access the values of lpPoints.
Each polygon has its own set of points.
Unlike
Polygon(), the nCount argument of PolyPolygon()
is the number of polygons you want to draw and not
the number of points.
The
lpPolyCounts argument is an array or
integers. Each member of this array specifies the
number of vertices (lines) that its polygon will
have..
Here
is an example:
void CExoView::OnDraw(CDC* pDC)
{
CPoint Pt[12];
int lpPts[] = { 3, 3, 3, 3 };
// Top Triangle
Pt[0] = CPoint(125, 10);
Pt[1] = CPoint( 95, 70);
Pt[2] = CPoint(155, 70);
// Left Triangle
Pt[3] = CPoint( 80, 80);
Pt[4] = CPoint( 20, 110);
Pt[5] = CPoint( 80, 140);
// Bottom Triangle
Pt[6] = CPoint( 95, 155);
Pt[7] = CPoint(125, 215);
Pt[8] = CPoint(155, 155);
// Right Triangle
Pt[9] = CPoint(170, 80);
Pt[10] = CPoint(170, 140);
Pt[11] = CPoint(230, 110);
pDC->PolyPolygon(Pt, lpPts, 4);
}
|
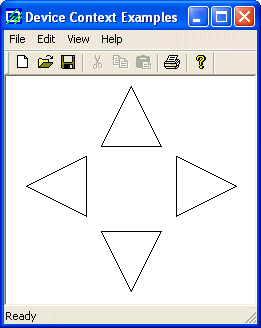 |
|