How to enable or disable the tooltip of each shape on
the canvas?
R. Call the
following method that defined within class file
FOGlobals.h:
// Enable canvas with tooltip showing or not.
FO_API_DECL void AFX_API FOPEnableToolTip(const BOOL &bEnable);
How to enable or disable the full feature of port
shape?
R. By
considering the memory size of the port shape, E-XD++ only
use simple port shape (Without the full property) at
start, with this state, you cann't change the port type,
port width or port height features, but if your
application still want to use other kind of port style,
you can call the following method to enable it (Defined in
class file FOGLobals.h:
// Enable full feature of properties of port.
FO_API_DECL void AFX_API FOEnablePortFullProp(const BOOL &bEnable);
How to enable or disable the auto scroll of canvas?
R. By
default, the canvas ships with auto scroll for canvas, but
if you want to disable this feature, call:
// Enable canvas auto scrolling or not.
FO_API_DECL void AFX_API FOPEnableAutoScrolling(const BOOL &bEnable);
How to enable the route style from left - right to top
- down?
R. By
default, when links route on the canvas, it shows from
left to right route style, but if you want to change it to
top - down style, call the following method:
// Enable link route from top or bottom.
FO_API_DECL void AFX_API FOPEnableRouteTop(const BOOL &bFromTop);
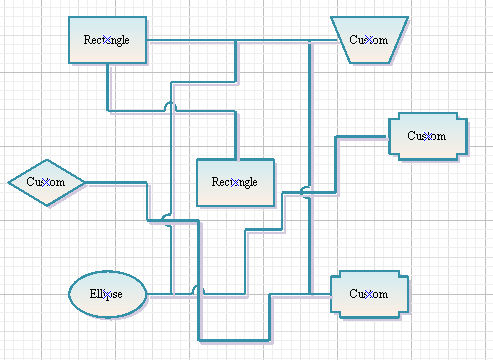
How to enable or disable the route feature of links?
R. With the
following method, you can enable or disable the route
feature of links:
// Enable link route or not.
FO_API_DECL void AFX_API FOPEnableLinkRoute(const BOOL &bEnable);
How to determine which kind of shape you take?
R. Mostly,
we can use IsKindOf(RUNTIME_CLASS), but we can also use
the following instead of:
#define HAS_BASE(pObj, class_name) pObj->IsKindOf(RUNTIME_CLASS(class_name))
How to use enable continue drawing feature?
R. By
default, after you drawing some on the canvas, it will be
changed to selection mode, but if you want to change it to
drawing - drawing - drawing ... state, please call the
following method to enable this:
// Enable continue drawing or not.
FO_API_DECL void AFX_API FOEnableContinueDraw(const BOOL &bEnable);
How to change the default width, height or type of the
port on the canvas?
R. You can
change the following values:
// Default port height
int m_nDefPortHeight;
// Default port width.
int m_nDefPortWidth;
// Default port type
int m_nDefPortType;
// Default port side
int m_nDefPortSide;
for
example:
gfxData.m_nDefPortHeight
= 15;
How to customize the drawing of Table's cell item?
R. Override
the following method that defined within class
CFOPVisualProxy:
// Drawing customized table cell.
virtual void DoDrawTableCell(CDC *pDC, const CRect &rcCell,const int &nRow,const int &nCol);
How to customize the pen style of tracking on the
canvas?
R. Override
the following method that defined within class
CFOPVisualProxy:
// Build track pen of the shape.
virtual CPen *BuildTrackPen(const int &nWidth);
Do something before any shape created?
R.
Override the following method of class CFODataModel, you
can do something before any shape created: // Call before create shape new shape,this method will be called after each new shape is created,
// You can override this method to do something before shape created.
// pShape -- the pointer of shape that created.
virtual void DoInitCreateShape(CFODrawShape *pShape);
Do something before link line is created?
R.
When any link is created on the canvas, the following
virtual method will be called (Defined in class
CFODataModel): // Add Link Shape
// m_drawshape -- Shape Type id start from FO_COMP_CUSTOM
// arPoints -- Init position of link shape.
// pFrom -- start link port shape pointer.
// pTo -- end link port shape pointer.
// nArrowType -- if being true,it has an arrow else it doesn't has an arrow.
// 0-None arrow.
// 1-One arrow at the end.
// 2- Two arrow,start arrow and end arrow.
virtual void AddLinkShape(UINT m_drawshape,
CArray<CPoint,CPoint> *arPoints,
CFOPortShape *pFrom = NULL,
CFOPortShape *pTo = NULL,
int nArrowType = 0); Override
this method to init the link line.
What's the quickest way to add hundreds of shapes to
canvas?
R.
Please call the codes like below: CRect rc = CRect(150,200,230,240);
int xy = 0;
CFODrawShapeList m_listUpdate;
for(xy = 0; xy < 300; xy ++)
{
CFORectShape *pReturn = new CFORectShape;
pReturn->AddRef();
pReturn->Create(rc,_T(""));
pReturn->UpdatePosition();
rc.OffsetRect(CPoint(10,10));
GetCurrentModel()->GetShapes()->AddTail(pReturn);
m_listUpdate.AddTail(pReturn);
pReturn->Release();
}
UpdateShapes(&m_listUpdate); Call
AddTail to add shape to the list of canvas at first, and
then call UpdateShapes one time, it will be very fast.
How to hide the ruler bar of canvas?
R.
Call the following method that defined in CFOPCanvasCore: // Show or hide ruler bar
// bVisible -- visible or not
virtual void ShowRulers(const BOOL &bVisible);
How to change the unit of the ruler by code?
R.
Call the following method that defined in CFOPCanvasCore: // Change the ruler unit.
// unit -- unit of the ruler mark,it must be one of the following value.
// enum FieldUnit
// {
// FUNIT_NONE = -1,
// FUNIT_INCHES, //0
// FUNIT_FEET, //1
// FUNIT_MM, //2
// FUNIT_CM, //3
// FUNIT_METERS, //4
// FUNIT_KM, //5
// FUNIT_YARDS, //6
// FUNIT_MILES, //7
// FUNIT_POINTS, //8
// FUNIT_TWIPS //9
// };
void SetRulerUnit(const FieldUnit &unit);
How to load a composite from resource?
R.
Call the code like below: CRect rc = CRect(0,0,100,100);
BOOL bFirst = FALSE;
CFOCompositeShape *pReturn = new CFOCompositeShape;
pReturn->AddRef();
pReturn->Create(rc,"");
pReturn->LoadFromResource(IDR_COMPSRES2);
CRect rcComp = CRect(0,0,0,0);
POSITION pos = pReturn->GetCompList()->GetHeadPosition();
CFODrawShape *pShape = NULL;
while(pos != NULL)
{
pShape = (CFODrawShape* )pReturn->GetCompList()->GetNext(pos);
pShape->UpdatePosition();
CRect rcPos = pShape->GetSnapRect();
if(bFirst)
{
rcComp = rcPos;
bFirst = FALSE;
}
rcComp = CFODrawHelper::GetMaxRectExt(rcComp,rcPos);
}
CPoint ptNew = CPoint(30,30);
CRect rcNew = CRect(ptNew.x,ptNew.y,ptNew.x + rcComp.Width(),ptNew.y+rcComp.Height());
pReturn->PositionShape(rcNew);
GetCurrentModel()->InsertShape(pReturn);
pReturn->Release();
pReturn = NULL;
How to load an emf file to canvas?
R.
Call the codes as below: CRect rc;
rc = CRect(20,20,200,100);
CFOWMFShape *pReturn = new CFOWMFShape;
pReturn->AddRef();
pReturn->Create(rc,_T(""));
pReturn->LoadImage("C:\\Temp\\test.wmf");
pReturn->SetObjectCaption(GetCurrentModel()->GetUniqueCaption(pReturn->GetType()));
pReturn->SetObjectName(GetCurrentModel()->GetUniqueName(pReturn->GetType()));
GetCurrentModel()->InsertShape(pReturn);
pReturn->Release();
How to add a rich text shape to canvas?
R.
Call the codes as below: CRect rcPos = CRect(20,20,200,200);
CFOPRichEditShape *pReturn = new CFOPRichEditShape;
pReturn->AddRef();
pReturn->Create(rcPos,this,"{\\rtf1\\ansi\\ansicpg1252\\deff0\\deflang2057{\\fonttbl{\\f0\froman\\fprq2\\fcharset0 Times New Roman;}{\\f1\\fswiss\\fprq2\\fcharset0 System;}}{\\colortbl ;\\red255\\green0\\blue0;\\red51\\green153\\blue102;\\red0\\green0\\blue255;}\\viewkind4\\uc1\\pard\\cf1\\i\\f0\\fs24 Inputted\\cf0\\i0 \\cf2\\b rich\\cf0\\b0 \\cf3 text\\cf0 !\\b\\f1\\fs20 \\par }");
GetCurrentModel()->InsertShape(pReturn);
pReturn->Release();
pReturn = NULL;
How to add a rectangle to the background of canvas?
R.
Call the codes as below: CFORectShape *pRect = new CFORectShape;
CRect rcPos = CRect(10,10,200,200);
pRect->AddRef();
pRect->Create(rcPos);
GetCurrentModel()->GetBackDrawComp()->AddShape(pRect);
pRect->SetBkColor(RGB(255,0,0));
pRect->Release();
Invalidate();
How to add a line shape to the canvas?
R.
Call the following codes: CArray<CPoint,CPoint> ptArray;
ptArray.Add(CPoint(20,20));
ptArray.Add(CPoint(120,90));
ptArray.Add(CPoint(200,40));
CFOLineShape *pReturn = new CFOLineShape;
pReturn->AddRef();
pReturn->Create(&ptArray);
CString strCaption = GetCurrentModel()->GetUniqueCaption(pReturn->GetType());
CString strName = GetCurrentModel()->GetUniqueName(pReturn->GetType());
pReturn->SetObjectCaption(strCaption);
pReturn->SetObjectName(strName);
GetCurrentModel()->InsertShape(pReturn);
pReturn->Release();
InvalidateShape(pReturn);
How to obtain the list of all shapes on the canvas?
R.
Call the following methods to obtain the shapes of
datamodel: // Obtain all the shapes on the canvas,it will return a pointer to the list of child shapes on the canvas.
CFODrawShapeSet * GetShapes() { return GetFormObjects(); }
// Obtain all the shapes on the canvas,it will return a pointer to the list of child shapes on the canvas.
void GetAllShapes(CFODrawShapeList &list);
// Get shapes count on the canvas.
int GetCompsCount() const;
// returns TRUE if the document has no shapes on the canvas.
BOOL IsEmpty() { return m_ShapeList.IsEmpty(); }
Call
the following methods to obtain the shapes within the
background of canvas: // get shape list
CFODrawShapeSet *GetCompList() { return &m_GroupList; }
// get shape count
virtual int GetCompCount() { return m_GroupList.GetCount(); }
How to obtain the list of shapes that selected?
R.
Call the following method that defined within class
CFOPCanvasCore: CFODrawShapeList *GetSelectShapes()
How to check which shape is hitted with mouse on the
canvas?
R.
Call the following method to do that: // Hit test, return the hit test shape pointer.
// point -- HitTest logical point.
virtual CFODrawShape * HitTest(
// Mouse hit point.
CPoint point
);
For port, please call: // Hit Test Port,ports are the shapes that link together.
// return the pointer of current shape's port shape.
// point -- HitTest logical point.
virtual CFOPortShape* HitTestPort(
// Mouse hit point.
CPoint point,
// Use extend border hit or not
const BOOL &bUseExt = TRUE,
// Hit expand value.
const int &nExp = 30
);
How to change the UI Unit of canvas?
R.
Call the following method that defined in class
CFODataModel: // Change the UI unit
// eUnit -- Unit type to be converted,it must be one of the following value:
// enum FieldUnit
// {
// FUNIT_NONE = -1,
// FUNIT_INCHES, //0
// FUNIT_FEET, //1
// FUNIT_MM, //2
// FUNIT_CM, //3
// FUNIT_METERS, //4
// FUNIT_KM, //5
// FUNIT_YARDS, //6
// FUNIT_MILES, //7
// FUNIT_POINTS, //8
// FUNIT_TWIPS //9
// };
void SetUIUnit(FieldUnit eUnit);
How to change the UI Scale of canvas?
R.
Call the following method to do that: // Default drawing scale,Default 1/1.
void SetUIScale(const FOPFraction& aScale);
How to enable each shape on the canvas has an unique
name?
R.
Call the following method that defined in class
CFODataModel: // Show with unique name and caption.
void EnableUniqueName(const BOOL &bUniqueName);
How to disable
the automatically link feature?
R.
When we move our mouse over any port (Read Corss within
any shape), we can start to click on it to draw link
immediate, but if we want to disable this feature, call
the following method (Defined in class CFODataModel): // Change to allow hole link mode,mostly,when we move mouse over the connection ports,we want to
// click the mouse to start creating links from this port right now.This method is defined for this kind
// of case,you can change the value of bAllow to switch to allow link or not.
void SetAllowHoleLink(const BOOL &bAllow);
How to obtain the size of the canvas?
R.
Call the method below (Defined in class CFODataModel): // Obtain the size of the canvas.
virtual CSize GetCanvasSize() const;
Which is the quickest way to rotate points?
R. Call the
following codes to rotate points: void
CMyShape::RotatePoints(int nAngle, double dStartX, double dStartY, LPPOINT cCtlPt, const int &nPtCount)
{
CFOMatrix* pMat = new CFOMatrix();
pMat->Move(-dStartX,-dStartY);
pMat->Rotate(nAngle);
pMat->Move(dStartX,dStartY);
CPoint ptx;
for (int j=0; j<nPtCount; j++)
{
ptx = cCtlPt[j];
pMat->ChangeValue(ptx);
cCtlPt[j] = ptx;
}
delete pMat;
pMat = NULL;
}
How to start timer within any shape on the canvas? R.
Call the codes as below: m_nTimerID = RegisterTimerEvent( this, 800 );
How to stop timer within any shape on the canvas?
R.
Call the codes as below: UnregisterTimerEvent( m_nTimerID );
m_nTimerID = -1;
How to update any shape within itself?
R.
Call the method as below: UpdateControl() ;
How to make shape to be cann't rotated?
R.
Call the codes as below: SetPolyObject(FALSE);
How to change the style of link bridge?
R.
Call the codes as below: gfxData.m_DefBridgeStyle
= FOPArc; There
are the following styles can be used: enum FOPBridgeStyle
{
FOPCap = 0,
FOPArc,
FOPSquare,
FOPSides2,
FOPSides3,
FOPSides4,
FOPSides5,
FOPSides6,
FOPSides7
};
.
How can I include the resources (*.rc file) if I have one
included already?
R: For VC6,
to include a resource file, for example, rores.rc
, go to View->Resource Includes.. and enter it in the
dialog box as shown:
For VS2005,
you can right click on an existing .rc file in the
resource view and select Resource Includes...
|